Given an integer array
Output: true
Explanation: Input array can be partitioned with two subsets [1, 5, 5] and [11].
Output: false
Explanation: Input array cannot be partitioned into two subsets.
Contents
Solution intution Solution using recursion Solution using recursion and memoization Solution using DP memoization and memory optimized
Below are two important decisions to make in the solution intution.
-
Compute the sum of elements from input array and if the value is an odd number,
then you can simply return
false , because it is not possible divide the input array into two subsets with equal sum.For example, consider the input array [1,2,3,5] and if you sum the elements it will come as 11, and it is not possible to split input array into two subsets with each sum as 5.5, so you can simply return false . -
If the sum of elements from input array is an even number, then calculate
sum/2 and check whether there is any subset array whose sum is equal to that.
For example [1, 5, 11, 5] will give sum as 22, and sum/2 is 11, and we can have subsets [1, 5, 5] and [11]. Another example [2,10] will give sum as 12, and sum/2 is 6, but there is no way we can have two subsets with sum as 6.
In this approach, we will compute the sum of elements from input array and check if if it even or odd,
if it is odd then return
-
In the recursion approach, we are going to check if there is any sub set sum available with following sub problems.
-
n-1 elements andsum . -
n-1 elements andsum - nums[n-1] .
-
- While solving the problem recursively, we can draw two base cases.
-
If the sum is reduced to 0, then a partition is possible, so return
true . -
If the number of eleemnts in array becomes empty, and the sum is stil greater than 0
or if the sum becomes less than 0, then return
false .
-
If the sum is reduced to 0, then a partition is possible, so return
- Below is an illustration how we are going to solve this recursively.
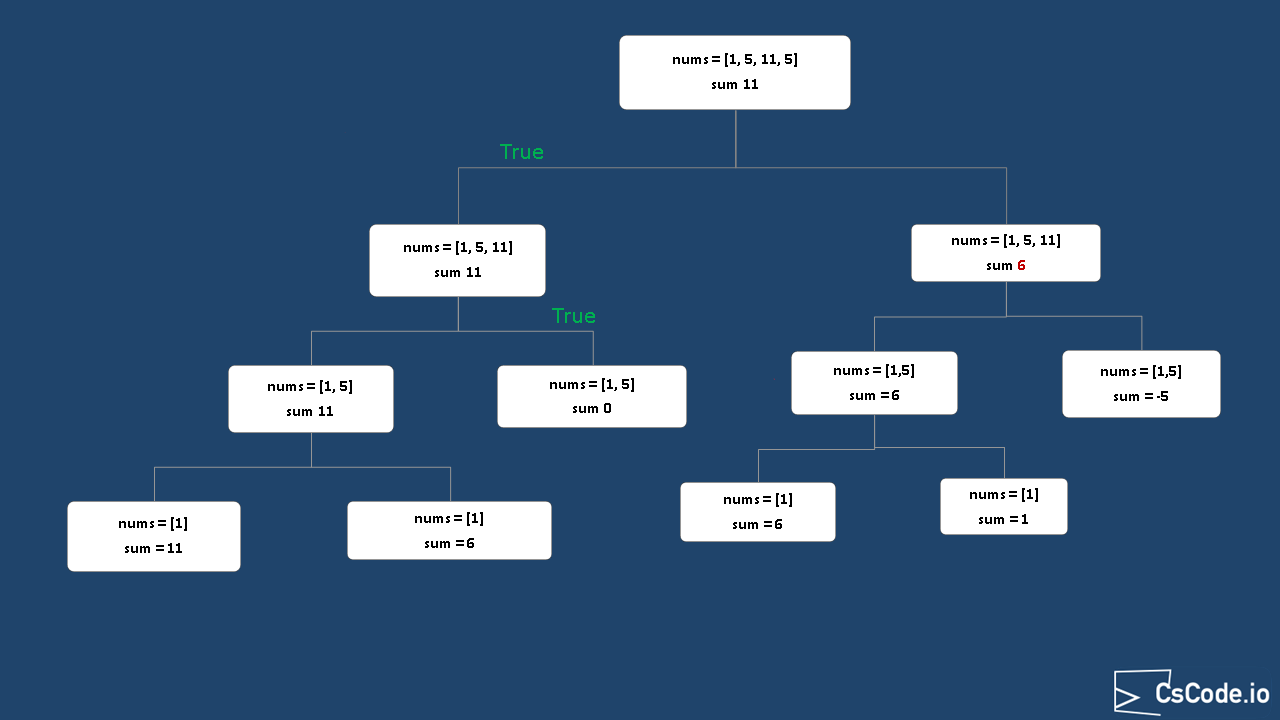
Complexity Analysis:
Time complexity: Above code runs in O(2n) time where
Space complexity: O(n2) as we are making recursive loops
As you can see in above solution, sub problems will be repeated (for example, circled sub problems in the below diagram), so we can cahe the sub problem answer and use it.
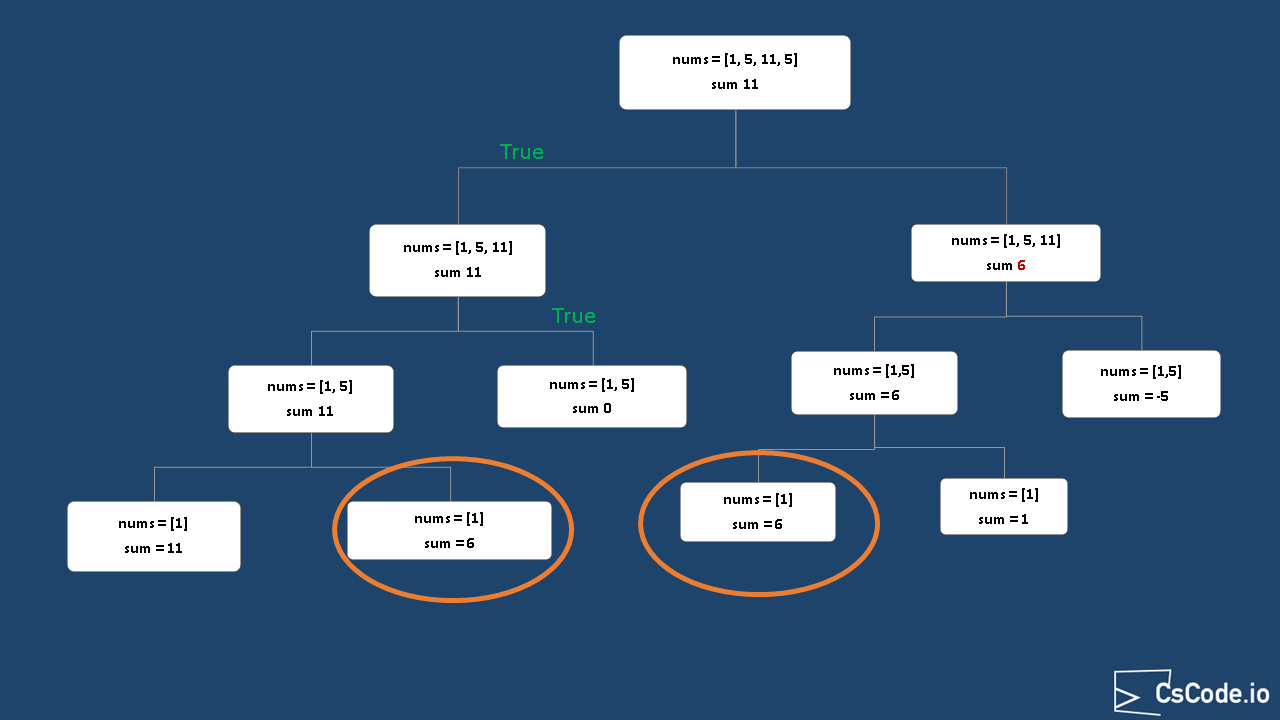
-
In this approach of memoization, we are going to cache the sub problem results with key as
current length of input array, sum and its result into aHashMap . - When the sub problem answer is found in the cache, we simply return that, if not then we call the function recursively and cache it.
Complexity Analysis:
Time complexity: Above code runs in O(sum * n) time where
Space complexity: O(sum * n) as there may be need for cache every answer.
In this approach, we are going to compute all possible sums for each number in the array and store them in a Set.
At the end return
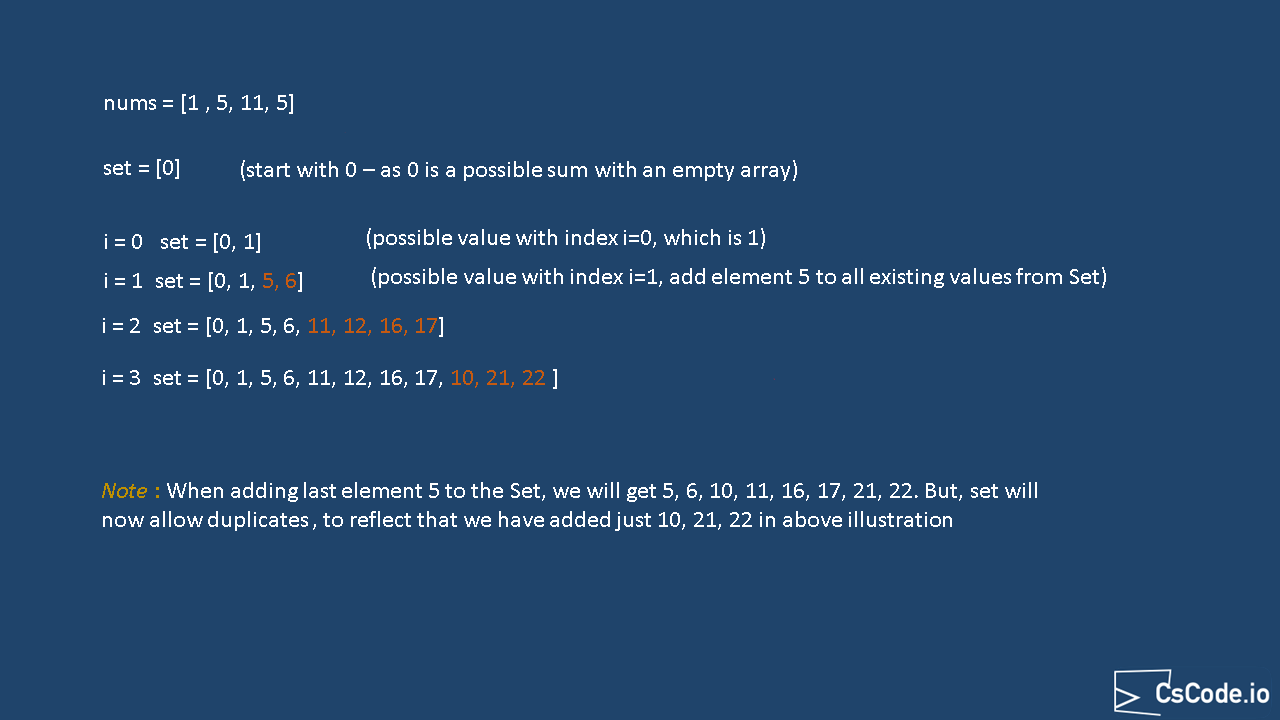
Complexity Analysis:
Time complexity: Above code runs in O(sum * n) time where
Space complexity: O(sum) as the max size of the set can be the sum.
Above implementations source code can be found at