Given a string
Output: "bab" or "aba"
Explanation: there are two longest palindromic substring in the input "bab" and "aba" and both are of length 3, so you return any one of them.
Output: "bb"
Explanation: longest palindromic substring is "bb".
Contents
Solution 1 - using brute force approach Solution 2 - expand around center
In this approach, we will generate all possible substrings, then for each substring check if that is a valid palindrome.
-
We will create a function
bruteForceApproach that takess as input string. -
Then, we will create all possible substrings using two
for loops.for(int i=0; i<s.length(); i++) { for(int j=i; j<=s.length(); j++) { String sub = s.substring(i,j); } } -
Create another helper function
isPalindrome that takes a Stringstr as argument and it checks whether the given string is a palindrome or not.- When checking whether input string is palindrome or not, there are two possibilities, when the string is an odd length string and when the string is even length string.
-
Create two variables
i andj to check characters on left and right side from the index where palindrome is explanding. -
When input string is odd length string, we take the mid index
mid = str.length() /2 , and seti=mid-1 andj=mid+1 , this is because when the string length is odd, then the mid index will be the center indexed element and we will checking whether it is palindromic string from the center.
For example, consider string "abcba", in this casemid = 5/2 which is 2, that is nothing but the center indexed character c. We wil be expanding around that center index, so we can seti=1 andj=3 . -
When input string is even length string, we take the mid index
mid = str.length() /2 , and seti=mid-1 andj=mid , this is because when the string length is even, there is no center indexed element for the palindrome. So, we have the check middle two characters and check whether they are same, and then expand around those two characters.
For example, consider string "abba", in this casemid = 4/2 which is 2, that is nothing but the right indexed element from the middle two elements. We wil be expanding around middle two elements, so we can seti=1 andj=2 . -
Once we have set
i andj variables, then check whether character at ith index and jth index are same or not, if they are same then decrementi and incrementj to continue checking other characters in the string.
If any of the characters on left and right are not same returnfalse otherwise returntrue at the end.
-
We will call this
isPalindrome for all substrings, and keep track of longest palindrome. - Finally return the longest palindrome substring found.
Complexity Analysis:
Time complexity: Above code runs in O(n3) time where
Space complexity: O(n) as we are creating substrings, and at given point of time we only have one substring.
In this approach, what we are going to do is, from every index in the string, we try to expand to the left and to the right and check whether the characters on both sides are same or not.
-
Create a helper method
expandAroundCenterHelper that takes parameters input strings , intl and intr left and right indexes to expand around.-
In the implementation of this method, create a variable
maxPalindrome to keep track maximum length palindrome found so far. -
We will then try to expand the palindromic substring using left and right indexes
l andr . -
While
l andr within the boundararies of input string's length, check character atl th index andr th indexes are same, that means we have found a palindrome, check the current length of palindrome usingr-l+1 (+1 is because the indexes starts with 0) and keep updating the variablemaxPalindrome if a maximum length palindrome found. -
If a palindrome substring is found, then decrement
l and incrementr and keep continuing as long as left and right indexes characters are same.
-
In the implementation of this method, create a variable
-
Now, call the helper method created above for both odd length palindromes and even length palindromes,
by supplying left and right indexes as
i, i for odd length palindromes, theni, i+1 for even length palindromes. Then, keep track of which palindrome is the largest one out of even / odd length palindromes returned.
For example, when you expand around center for input string "babad", it will produce below output.
Below diagram only shows odd length palindromes, but the logic for even length palindromes is same,
expect you start with two characters from indexes
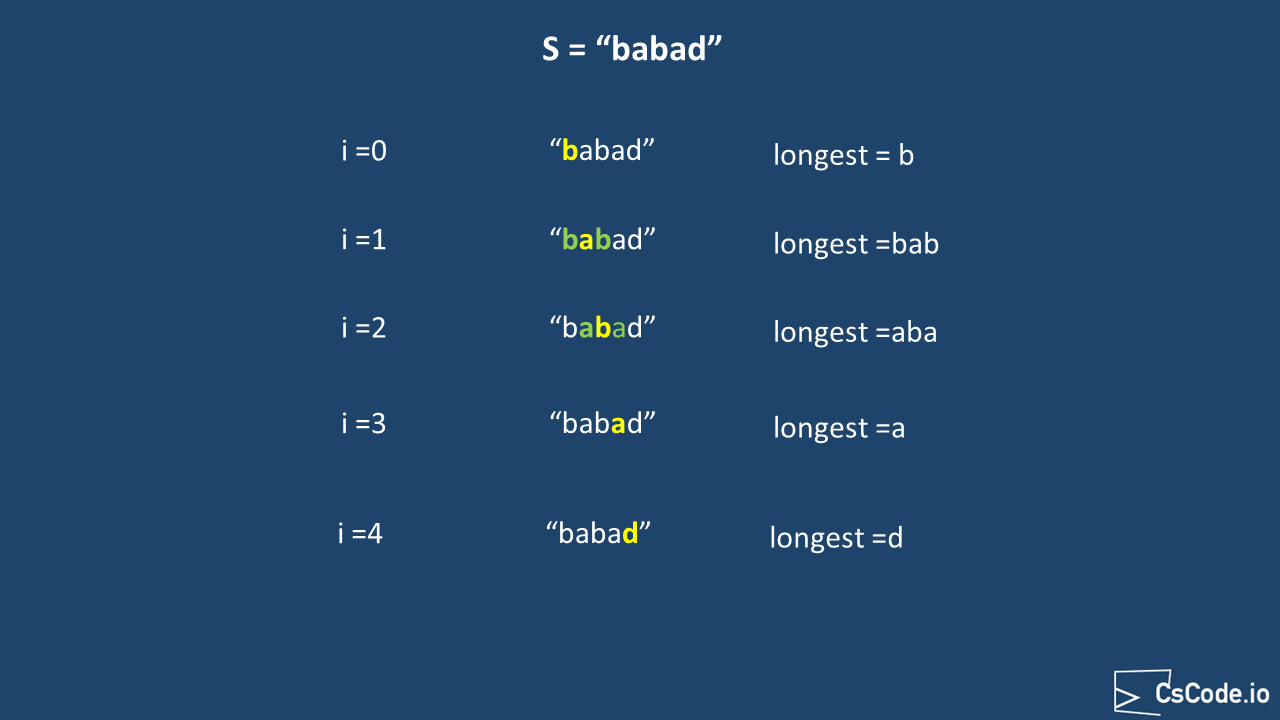
Complexity Analysis:
Time complexity: Above code runs in O(n2) time where
Space complexity: O(n), since we have to keep track of maximum length palindrome substring.
Above implementations source code can be found at