You are given a 0-indexed 2D array
Both robots initially start at
At the start of the game, the first robot moves from
The first robot wants to minimize the number of points collected by the second robot. In contrast, the second robot wants to maximize the number of points it collects. If both robots play optimally, return the number of points collected by the second robot.
Output: 4
Explanation: The optimal path taken by the first robot is shown in orange, and the optimal path taken by the second robot is shown in blue.
The cells visited by the first robot are set to
The second robot will collect 0 + 0 + 4 + 0 = 4 points.
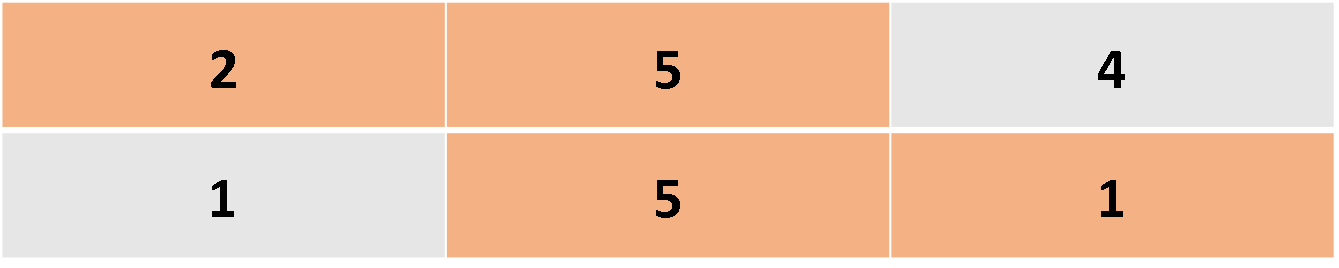
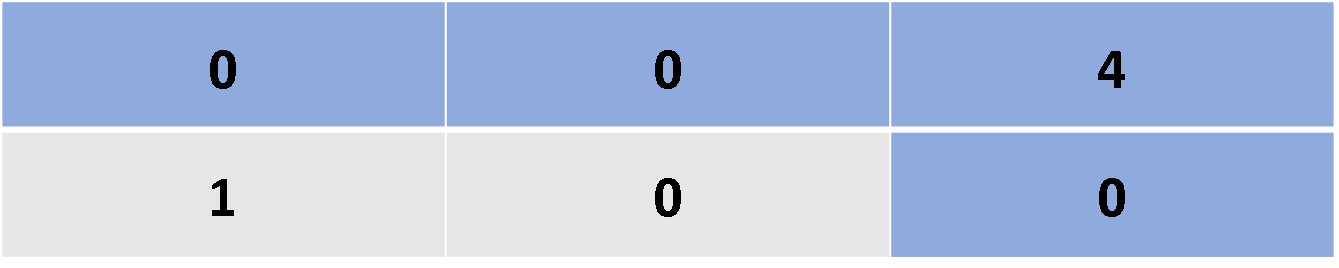
Output: 4
Explanation: The optimal path taken by the first robot is shown in orange, and the optimal path taken by the second robot is shown in blue.
The cells visited by the first robot are set to 0.
The second robot will collect 0 + 3 + 1 + 0 = 4 points.
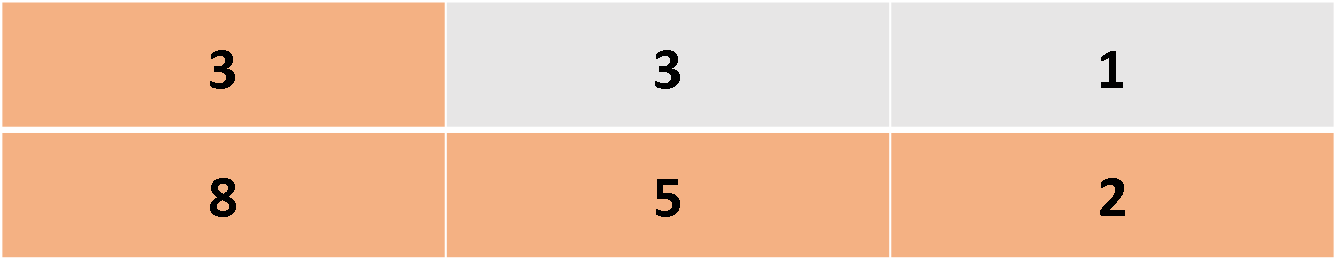
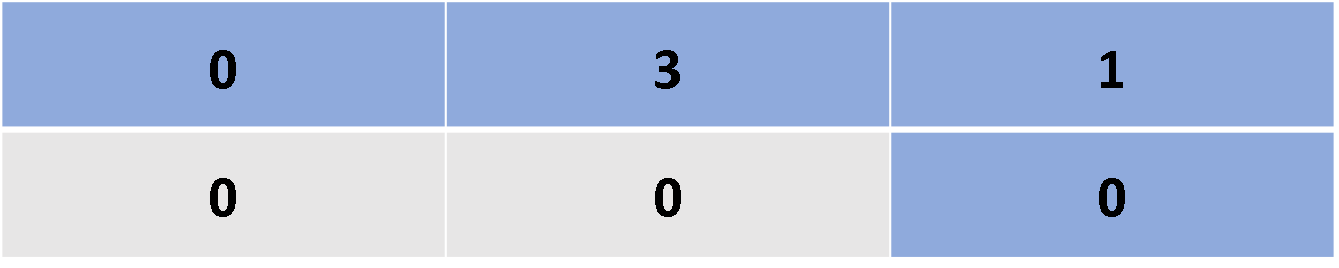
Output: 7
Explanation: The optimal path taken by the first robot is shown in orange, and the optimal path taken by the second robot is shown in blue.
The cells visited by the first robot are set to 0.
The second robot will collect 0 + 1 + 3 + 3 + 0 = 7
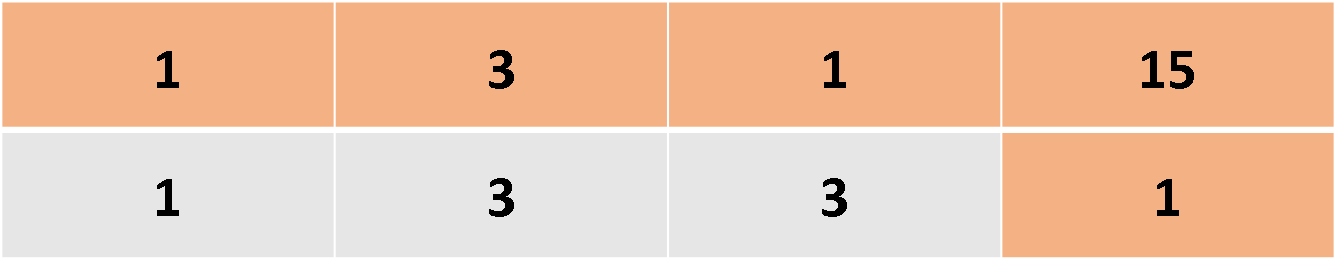
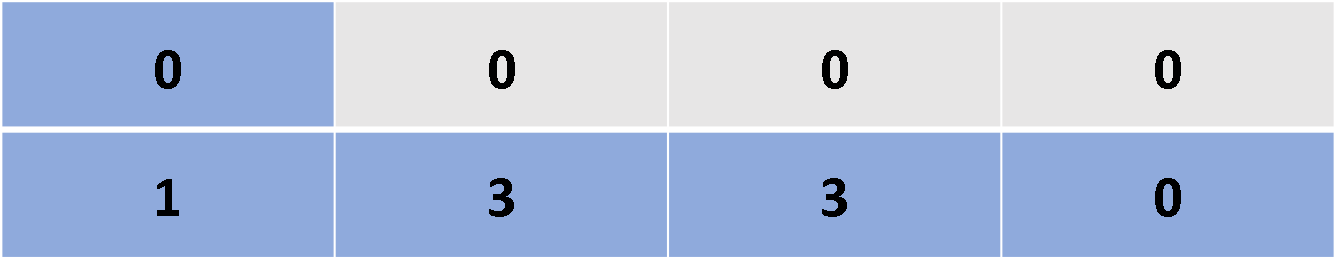
Contents
Solution 1 - Using prefix/postfix, calculate the sum left for second robot to collect
As per the problem statement, one of the key point to remember is that the each robot may move right or down, that means if the robot comes down it has to continue in the same row.
In this approach, we will track the minimum value that the first robot should aim for at each step when it is moving, this minimum value when tracked by first robot, it will ensure that the maximum can be achieved by second robot is as small as possible.
Implementation steps:
-
We will first compute the total sum of 1st row, call it
totalSum . -
Next, at each column
i , from the first row compute what would be left for second robot if that celll is taken by first robot. that istotalSum - cell value . -
Then compute the maximum between the accumulated sum of the second row and the remaining sum of the first row and store it
in a variable, call it
result . -
In each step, track minimum value among
result variable computed in each iteration, that will be the maximum that second robot can collect, after first robot tried its best to minimize second robot's score.
Example:
Lets look at an example with input array
-
First, let's compute the total of 1st row, which will be
1 + 3 + 3 + 15 = 22 . -
Next, for every column
i do the following:-
For column
1 , compute how much left for second robot if its comes down at this column,total - cell value =>22 -1 = 21 , and accumulated sum for second row is 0bottomRowAccumulated = 0 (because if first robot comes down to bottom row, at this column this cell at bottom row will not be collected by second robot), then compute themax (total - cell value, bottomRowAccumulated) =>max(21, 0) = 21 . Now updatebottomRowAccumulated with bottom row value for next iterationbottomRowAccumulated = 1 . -
For column
1 , compute how much left for second robot if its comes down at this column,total - cell value =>21 -3 = 18 , and accumulated sum for second row isbottomRowAccumulated = 1 , then compute themax (total - cell value, bottomRowAccumulated) =>max(18, 1) = 18 . Now updatebottomRowAccumulated with bottom row value for next iterationbottomRowAccumulated = 1+3 = 4 . -
For column
2 , compute how much left for second robot if its comes down at this column,total - cell value =>18 -3 = 15 , and accumulated sum for second row isbottomRowAccumulated = 4 , then compute themax (total - cell value, bottomRowAccumulated) =>max(15, 4) = 15 . Now updatebottomRowAccumulated with bottom row value for next iterationbottomRowAccumulated = 4+3 = 7 . -
For column
3 , compute how much left for second robot if its comes down at this column,total - cell value =>15 -15 = 0 , and accumulated sum for second row isbottomRowAccumulated = 7 , then compute themax (total - cell value, bottomRowAccumulated) =>max(0, 7) = 7 . Now updatebottomRowAccumulated with bottom row value for next iterationbottomRowAccumulated = 7+1 = 8 .
-
For column
-
At each step above, if we track the minimum of
max (total - cell value, bottomRowAccumulated) , it will be 7 and that is our answer for second robot.
Complexity Analysis:
Time complexity: Above code runs in O(n) time where
Space complexity: O(1)
Above implementations source code can be found at