You are given an integer array
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return the maximum amount of water a container can store.
Notice that you may not slant the container.
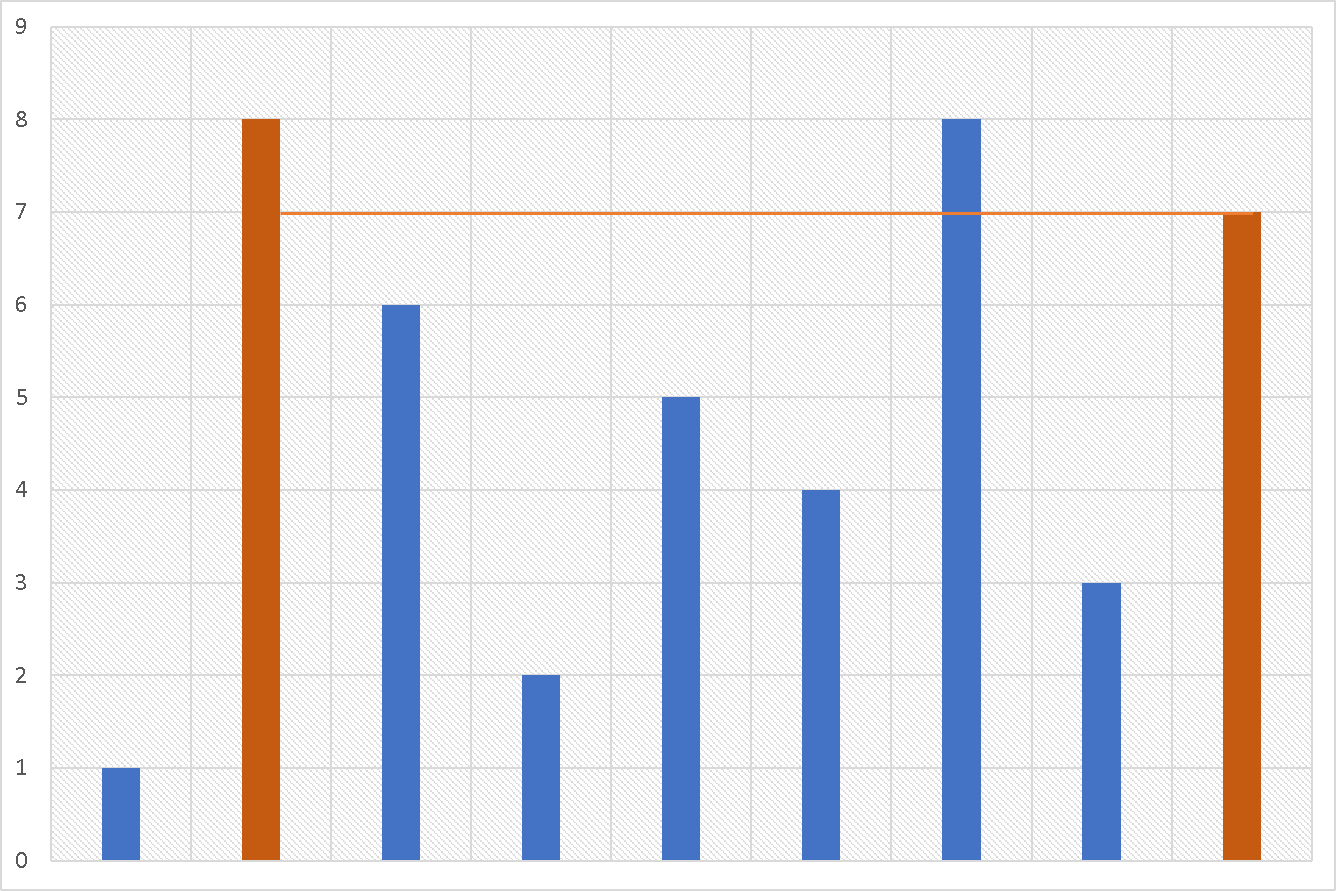
Output: 49
Explanation: The above vertical lines are represented by array [1,8,6,2,5,4,8,3,7].
In this case, the max area of water (two red poles connected with a line) the container can contain is 49.
Output: 1
Constraints:
-
n == height.length -
2 <= n <= 105 -
0 <= height[i] <= 104
Contents
Solution 1 - Using two pointers, compute area between two heights and keep track of maximum area
In this approach, we are going to use two pointers on both sides of
Implementation steps:
-
First, we will two variables
left , andright to point both ends ofheight array, and another variablemaxArea to keep track of maximum area. -
Then, we will compute the
area between the heights at left and right pointers, usingarea = MIN(heightleft, heightright) * width ( here, width is the difference between two pointersright andleft ). -
Now, compare
area withmaxArea , if current area is greater, then updatemaxArea with current area. -
Now, when updating
left or right pointers, since we can hold the water until the minimum height among two heights, move the smallest one inorder to check if there is any bigger height:-
If the
height[left] is smaller thanheight[right] , then incrementleft pointer. -
Otherwise, decrement
right pointer.
-
If the
-
Finally, return
maxArea .
Complexity Analysis:
Time complexity: Above code runs in O(n) time, where
Space complexity: O(1).
Above implementations source code can be found at